This is a short article on how to make electron app from website. Electron io is a library capable of turning electron js to cross platform desktop applications.
TLDR:
- Clone
git clone https://github.com/nomadicsoft/electron-webview-quickstart.git
- Run
npm install
- Change link in main.js:
mainWindow.loadURL('https://nomadicsoft.io/')
- Run
npm pack
- Resulting .exe and .msi will be in the
dist
folder
Fastest way to have a meaningful desktop app which actually does something is to use electron js and just open a website inside a webview:
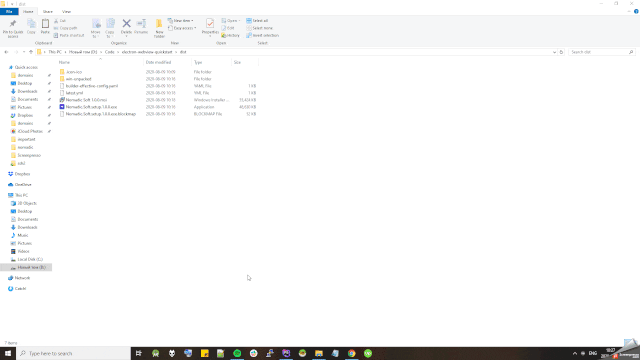
All in all it's a pretty simple and straightforward task, just a few notes along the way.
You can start by cloning https://github.com/electron/electron-quick-start or https://github.com/nomadicsoft/electron-webview-quickstart which is essentially the same thing but already prepared to run an external url in a resulting window instead of the js app you would compile yourself.
So you can get the following code:
const {app, BrowserWindow} = require('electron') const path = require('path') function createWindow () { const mainWindow = new BrowserWindow({ width: 1024, height: 768, webPreferences: { preload: path.join(__dirname, 'preload.js') }, title: 'Nomadic Soft' }) mainWindow.loadURL('https://nomadicsoft.io/') mainWindow.setMenu(null) // Remove this line to have menu mainWindow.on('page-title-updated', (evt) => { evt.preventDefault(); }); } app.whenReady().then(() => { createWindow() app.on('activate', function () { if (BrowserWindow.getAllWindows().length === 0) createWindow() }) }) app.on('window-all-closed', function () { if (process.platform !== 'darwin') app.quit() })
I removed a default menu, because it doesn't really make sense:
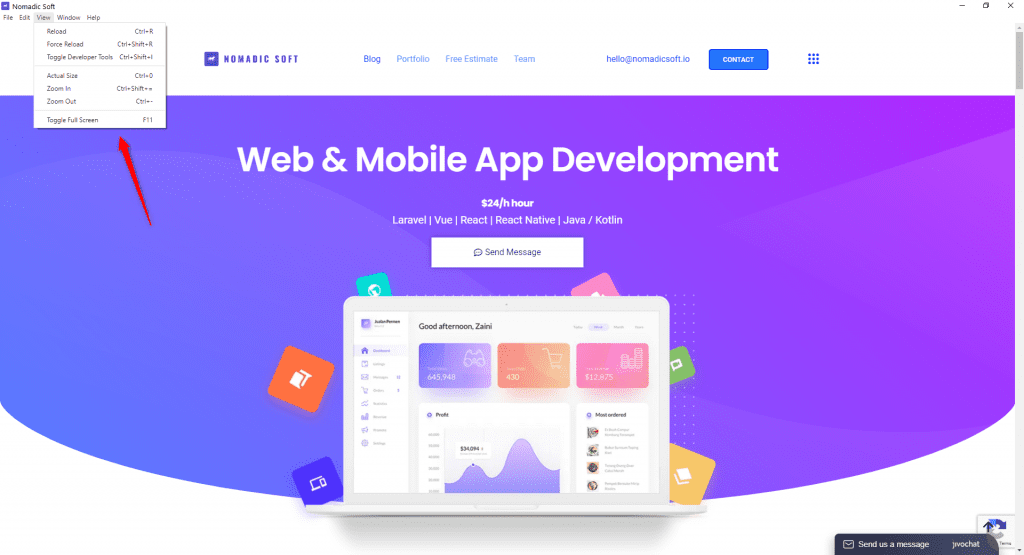
By default, electron would generate quite a bunch of unneeded stuff in the dist folder which is required for your app to run, which is not really nice and shareable.
Luckliy there are two packages to compile your app into a single file electron-builder
and electron-packager
. I liked the first one more, it has better documentation, more options and easier to work with https://www.electron.build/
So you just fire: npm run pack
and you're good. (assuming you have windows for other platforms you would need to change command signature in package.json
)
The electron app you have is fully functional except a few window functions: alert, confirm, prompt. So avoid those.
Code: https://github.com/nomadicsoft/electron-webview-quickstart
This article shows you how to create a minimal electron app. At the same time electron framework is capable for creating complex cross platform desktop apps