How to Set Up the Repository for Dependency Injection
Setting up the repository for Nextjs dependency injection is a crucial first step. It enhances your Next.js development. Start by creating a new GitHub repository tailored to your project requirements. Make sure you organize the repository well. Use separate folders for class components, services, and utilities, rather than creating mixing them together. This will help streamline the implementation of dependencies later on. Start the repository with a README. The README details the purpose and project setup. Consider adding a license file to ensure proper attribution and permissions for usage. Don't forget to set up version control using Git. It tracks changes well and helps you work with team members. Set a clear foundation for your project repository from the start. This paves the way for smooth use of DI. It also boosts the code's maintainability.Steps for Creating Dependencies
Creating dependencies for your Next.js application can be easy. You just need a few key steps. List the external packages or modules your project needs. Your app needs APIs, utilities, or custom services. Define what is essential for them. Use TypeScript to declare and manage these dependencies. Do so in a structured and type-safe way. This ensures better code organization. It helps stop runtime errors. They are related to missing or incorrect dependencies. Then, establish clear interfaces for each dependency to promote loose coupling between components.
Define contracts. They specify how your app's parts interact with software dependencies. This enhances flexibility and maintainability. Utilize tools like TSyringe to facilitate dependency injection implementation in an efficient way. Properly set up and configureconst
containers and registrations. Then, managing dependencies becomes easy in your Next javascript project.
Importing Necessary Packages
When starting a project for DI in Nextjs with TS, one key step is to import the needed packages. These packages will enable us to implement DI effectively within our application. To begin, make sure to install the required packages like tsyringe through npm install or yarn. The library is vital for managing dependencies. It injects them into our code easily. By importing these packages correctly.
We set up the foundation for strong and maintainable code. It lets us use the dependency inversion principle well. It will improve our Next structure. Selecting the right packages can greatly affect your project. Don't forget that.Take time to research and choose wisely based on your specific project requirements. Prop selection and management is crucial in maintaining a well-structured and efficient application.
Implementing Dependency Injection with TypeScript
Using DI with TypeScript in Nextjs apps is powerful. It helps manage dependencies and improve code maintainability through injectable components. By using DI, you can easily swap out implementations. It will also improve testability and follow SOLID principles.
TS, developed by Microsoft, has a strong typing system. It lets you catch errors at compile time and ensure type safety in your app. You set up DI container and register dependencies using libraries like TSyringe. This gives you a clear structure for managing your dependencies. This reduces coupling between parts. It also allows for easier scaling as your project grows. Using DI to implement IoC separates concerns in your code. With good dependency injection and automatic injections, you can create more modular and reusable components. They are easier to maintain over time. This approach makes code cleaner. It helps team members working on the same project collaborate smoothly.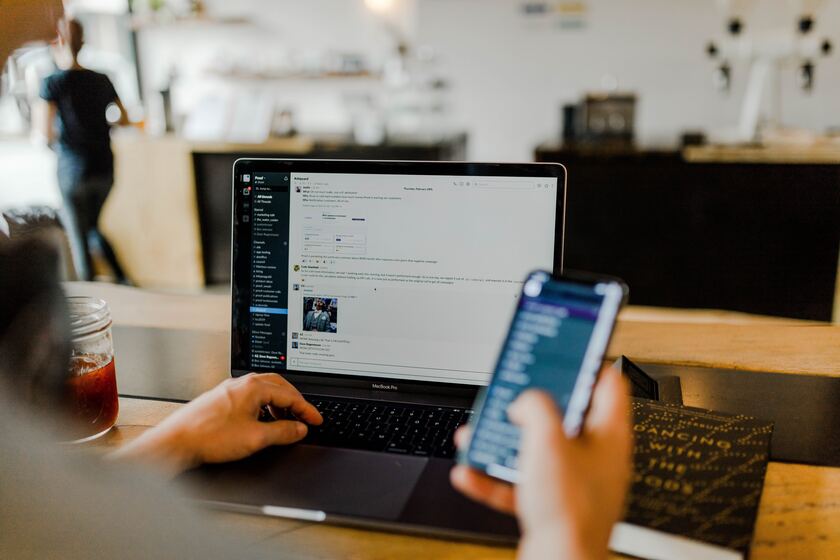
Exploring Code Structure and Dependencies
When you delve into a project's code and its dependencies, it's like embarking on a journey. You travel through interconnected paths of logic and function. Each file and module is crucial in the architecture. They create a web of interdependencies. Exploring these connections can reveal insights. They show how parts of the app interact. It offers a glimpse into the design choices. These choices shape how the application behaves and performs. By dissecting the codebase, creators of Next.js can understand it better. They can see its inner workings. Dependencies act as building blocks that enable various features to function harmoniously together. They are the backbone of the app. They provide vital resources and functions.
They are key variables for smooth operation. Understanding these dependencies is key to maintaining scalability and flexibility within the project. In essence, navigating code structures and dependencies is like solving a complex puzzle. Each piece contributes to the bigger picture. Variables are a key part of software development. It needs careful attention to detail and an analytical mindset.Understanding the Package.json Configuration
In a Nextjs app with TypeScript, managing dependencies is key. Understanding the package.json
config is crucial. This file serves as the entry point for defining project metadata and dependencies. In the package.json
, you can specify many scripts. These are for building, testing, and running your application. One key part of package.json
is declaring all needed dependencies. They use specific versions to ensure compatibility and stability. Also, you can export custom commands.
Exploring the Folder and File Structure
When we dive into the folder and file structure of our Next js app, we find a well-organized layout.
- Code Readability and Maintainability: Organizing code into directories enhances its readability and maintainability, making it easier for developers to understand and work with.
- "pages" Directory for React Components: The "pages" directory contains React components, facilitating seamless routing within the application.
- "Components" Directory for Reusable UI Elements: Reusable UI elements are stored in the "Components" directory, promoting modularity and reusability throughout the project.
- "utils" Folder for Helper Functions: The "utils" folder houses helper functions that can be shared efficiently across different parts of the project, promoting code sharing and reducing duplication.
- Functionality Files and Configurations: Further within the directory structure, functionality files such as "tsconfig.json" for TypeScript configurations are found, streamlining development with TypeScript.
- Version Control Management: Configuration files like ".gitignore" are included to specify which files should be skipped from version control, ensuring a clean and organized repository.
- Specific Purpose for Each File: As developers navigate through directories, each file serves a specific purpose, contributing to the overall functionality and structure of the project.
Using TSyringe for Dependency Injection
When it comes to managing dependencies in a Next js app with TypeScript, TSyringe is a powerful tool. It simplifies the process of DI. TSyringe lets you register and resolve dependencies in your app. It promotes cleaner code and better organization. You can use TSyringe's constructor injectable features. They improve the testability and maintainability of your Next js project. With TSyringe, you can handle inversion of control well.
It does this by decoupling components from their dependencies. This approach improves your code's flexibility. It also makes it easier to replace or update dependencies. Adding TSyringe to your workflow makes it easier to manage dependencies in your Next apps. Consider incorporating a GraphQL Client for seamless integration with GraphQL API and efficient data fetching.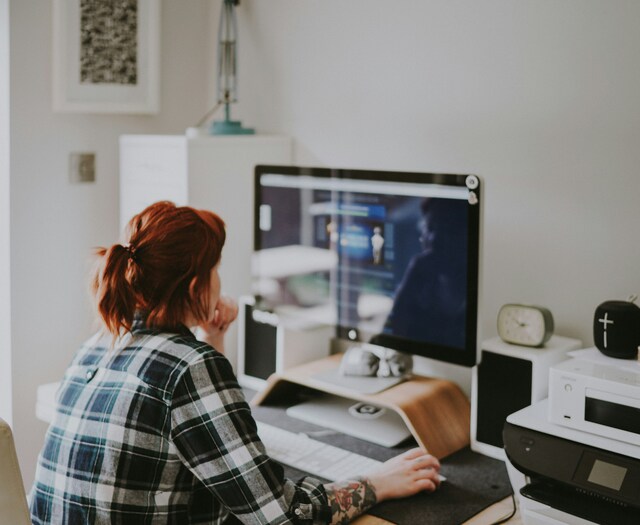
Deploying the Next.js App on Vercel
You have added Next.js dependency injection to your Nextjs app. Now, it's time to deploy it on Vercel for the world to see. Deploying on Vercel is a smooth process that ensures your app is live and accessible in no time. Just commit your changes to GitHub. Then, watch Vercel trigger the deployment. The seamless integration between Git repositories and Vercel makes deploying a breeze. Once deployed, you can inspect the latest commits directly from Vercel's dashboard. This allows you to track changes and monitor the status of your app effortlessly. With your Next now live on Vercel, users can access it with just a simple URL link. Deploy on Vercel for seamless hosting and instant scaling capabilities. The convenience of deploying on Vercel means your app is ready for use without any hassle or delay.Committing Changes and Triggering Deployment
You have made the needed changes to your Next and set up all dependencies. Now, it's time to commit those changes to your repository. Committing code ensures that your project history is well-documented. It allows easy tracking of changes over time. After you commit your changes. Then, deploying on platforms like Vercel will make your updated app go live. Users can then access it. This step is crucial in ensuring that the latest language version of your app is available for use by visitors.
Follow this process carefully. It will let you keep a smooth workflow when updating and deploying changes to your Next project. Remember, being consistent in making query updates and triggering deployments improves your development cycle. It makes it more efficient and reliable.Inspecting the Latest Commits
We inspected the latest commits in the repository. They give us valuable insights into our project's evolution. Each commit tells a story of progress, with changes and improvements enhancing its extensibility.
- Insight into Development Journey: Examining commits is like peering through a window into the development journey, revealing how ideas evolved into tangible code.
- Bug Fixes and Feature Additions: By reviewing commits, developers can observe bugs being fixed, new features being added, and optimizations being made over time.
- Facilitating Collaboration: The transparency provided by commit histories helps teams collaborate effectively, ensuring everyone is informed about project updates and progress.
- Navigation of Repository Files: Navigating through repository files allows for easy identification of errors or issues that may have occurred during development, aiding in troubleshooting and debugging efforts.
- Checkpoint for Project Health: Inspecting commits serves as a checkpoint for assessing the project's health and stability, clarifying the progress that has been made and the current state of the codebase.
It shows what still needs attention. Each commit represents a step closer to our goals for the Next app, including modeling TS DI with property injections.
Getting Started with the Deployed Next.js App
So, you've successfully deployed your Next js app on Vercel - congratulations! It's time to dive into exploring the live version of your project. Navigate to the provided URL and witness your app come to life in the online realm. Click through the different pages and functionalities to see everything in action. Test out the features, buttons, and interactions. Make sure that all of your app works on the live platform.
Take note of any issues or bugs that may arise during your exploration. This is a great chance to refine your app. You can make it better for users. Share the link with friends, colleagues, or testers for their feedback. Getting diverse viewpoints can help you find areas to improve. It will also improve the usability of your Next js app.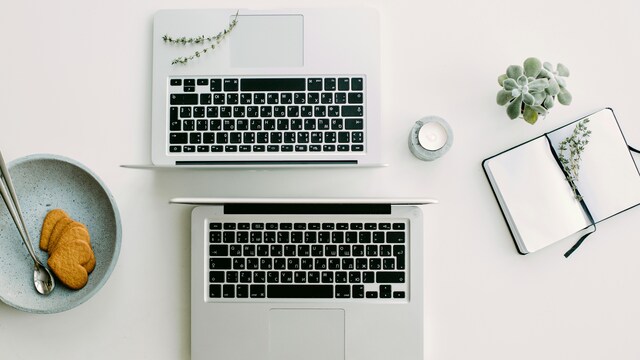
Implementing Dependency Injection and Inversion of Control in Next.js
Using Dependency Injection and Inversion of Control in Nextjs allows for more flexible. It also allows for more maintainable code. By separating components from their dependencies. We can easily swap out implementations. This is done without changing the core logic. This approach promotes reusability and testability. It does so by letting us mock dependencies in unit tests. In Next.js, we can use DI. It provides components with needed dependencies through inversion of control. This helps in separating concerns and promoting a cleaner architecture. We register dependencies in containers. This sets clear boundaries between parts of our app. Following these principles ensures our code stays scalable. It also keeps it easy to extend. It keeps our app organized. And, it keeps it manageable. By using DI in Next, developers can improve their apps. It also makes future maintenance simpler.
Utilizing DI for React Components
Using Dependency Injection (DI) in ReactJS components is a powerful way to improve code. It makes it more reusable and maintainable. By injecting dependencies into components, we can easily swap out implementations without modifying the component. This leads to more flexible and scalable code.With DI, ReactJS components become more modular and easier to test. We decouple dependencies from the component logic, creating a stack of independent modules using the decorators software design pattern rather than relying solely on component lifecycle. This improves separation of concerns and cleans our codebase.
Aspect | Description |
---|---|
Dependency Injection | Incorporate DI framework/library such as InversifyJS or TSyringe to inject dependencies into React components. |
Modular Components | Break down components into smaller, reusable modules, allowing for easier management and testing. |
Decoupled Logic | Decouple component logic from its dependencies, promoting cleaner and more maintainable code. |
Testability | Improve testability by injecting mock dependencies during unit testing, facilitating easier and more comprehensive testing of individual components. |
Separation of Concerns | Enhance separation of concerns by separating dependency resolution from component implementation, making it easier to understand and maintain the codebase. |
Scalability | Enable scalability by allowing components to be easily extended or modified without directly affecting their dependencies, thus promoting flexibility and adaptability. |
Understanding SOLID Principles for Next.js Development
In Next dev, you must understand the SOLID principles. They are crucial for writing clean architecture and maintainable code. Each letter in SOLID represents a fundamental principle that guides software design. The Single Responsibility Principle (SRP) is a great starting point. It says each component should have only one reason to change, ensuring that you don't overload a component without clear separation of concerns.
This helps keep your backend codebase modular and easy to maintain. The Open/Closed Principle (OCP) encourages you to design components. They should be open for adding to, but closed to changing. Tag your components appropriately to facilitate this process.By adhering to OCP, you can easily add new features without altering existing code. LSP says objects of a superclass should be replaceable.
You should be able to replace them with objects of its subclasses. This won't break the program. ISP suggests breaking interfaces into smaller ones. They should be specific. This way, clients only need to implement what they use. DIP promotes decoupling high-level modules from low-level code. It uses abstractions that depend on abstractions. They enable flexibility and easier testing in Next js. Additionally, instantiate singleton to ensure a single instance throughout the application.
Registering Dependencies and Containers
In Next apps, dependency injection in Next.js is key. Registering dependencies and containers is crucial. They manage the flow of data in your app. Map out which components need certain dependencies. This ensures each part of your app works together. Registering dependencies involves saying which classes or instances should be injected.
You specify where they should go. This process allows more flexibility and scalability in your programming code. It makes it easier to swap out dependencies or add new ones as needed. Containers act as centralized repositories where all dependencies are stored, managed, and instantiated. They link classes without tightly coupling parts of your app. They use JavaScript frameworks for DI. This promotes better organization and maintainability. By registering dependencies well and using containers effectively, you can streamline development, making it more robust. This will also improve the whole layout and speed of your Next app. Consider incorporating SWC for enhanced compilation speed if applicable to your project. Consider integrating Vue for enhanced frontend capabilities.Improving Testability and Maintainability with Dependency Injection
You used Dependency Injection in your Nextjs app with TS. It organized your code and improved testability, maintainability, and code reusability. This practice makes unit testing easier. It also reduces coupling between components. It makes swapping out implementations simpler, allowing for easy replacement of class instances. Using DI promotes a more modular codebase.
It is also more flexible and follows SOLID principles. Use the sample project on GitHub as a reference. It will help you add DI and Inversion of Control to your Next js apps. Registering dependencies will help. Using containers well will, too. Both will streamline your dev while ensuring scalability and ease of maintenance.
Use DI in Next with advanced TypeScript. It will improve your projects and make future updates or additions easier. Keep exploring the best software development practices. This will help you improve your skills and deliver high-quality solutions.