HOW CAN I CHECK THE REACT VERSION IN MY PROJECT?
Checking the React-version in your project is a vital step for effective development. There are several methods to do this, each with its own advantages. The easiest way is through the package.json file.
This file contains all your project's dependencies and their respective versions. Simply open it up and look for "react" under the dependencies section. If you prefer command line tools, you can easily check using npm or yarn commands. Run npm list react
or yarn list react
.
console.log(React.version)
in your JS files. Each approach gives a quick look at your app's behind-the-scenes. It helps keep everything organized and up-to-date.
USING PACKAGE.JSON TO FIND REACT VERSION
To check the React-version in your project, start with the package.json
file. This file is a treasure trove of information about your project's dependencies. Locate package.json
at the root of your project directory. Open it using any code editor or text viewer.
- Look for the "dependencies" section, this lists all packages used in your application.
- Within that section, you should find an entry for "react".
- The number next to it indicates which version you’re currently using. If you're curious about related libraries like "react-dom", they'll be listed here too, along with their versions.
CHECKING REACT VERSION VIA COMMAND LINE
To check the current React version via the command-line, open your terminal. Then, navigate to your project directory. This is where all of your dependencies are stored.
- Navigate to the Project Directory: Ensure you are in the correct project folder using your terminal or command prompt.
- npm Users: Execute the cmd
npm list react
to display the installed React-version and its location within thenode_modules
folder. - Yarn Users: Execute the cmd
yarn list --pattern react
to display similar information about the installed React version.
VERIFYING REACT VERSION IN YOUR JAVASCRIPT CODE
To verify the React-version in your JavaScript code, check the version object. If you've already imported React, log React.version
to the console. This line of code will return a string representing the current-version of React being used. It's a quick, simple method. It lets you check the running version in real-time.
What's The Difference between Comparing React and React Native Versions?
When it comes to checking versions, React and RN serve different purposes. React is primarily a library for building web applications. If you want to check its versioning, it focuses on improvements in rendering efficiency and component-based architecture. You’ll often check this, along with your webpack configuration, when ensuring your web app runs smoothly across web browsers. RN is geared towards mobile app development. It allows developers to create native apps using an open-source JavaScript library and React's principles for building rich UI components. Version checks often relate to compatibility with iOS or Android. Understanding these differences helps you choose the right tools for your project needs. Each environment has unique updates that can affect performance and latest features significantly. So, knowing which version you're using can save time and help troubleshoot issues later.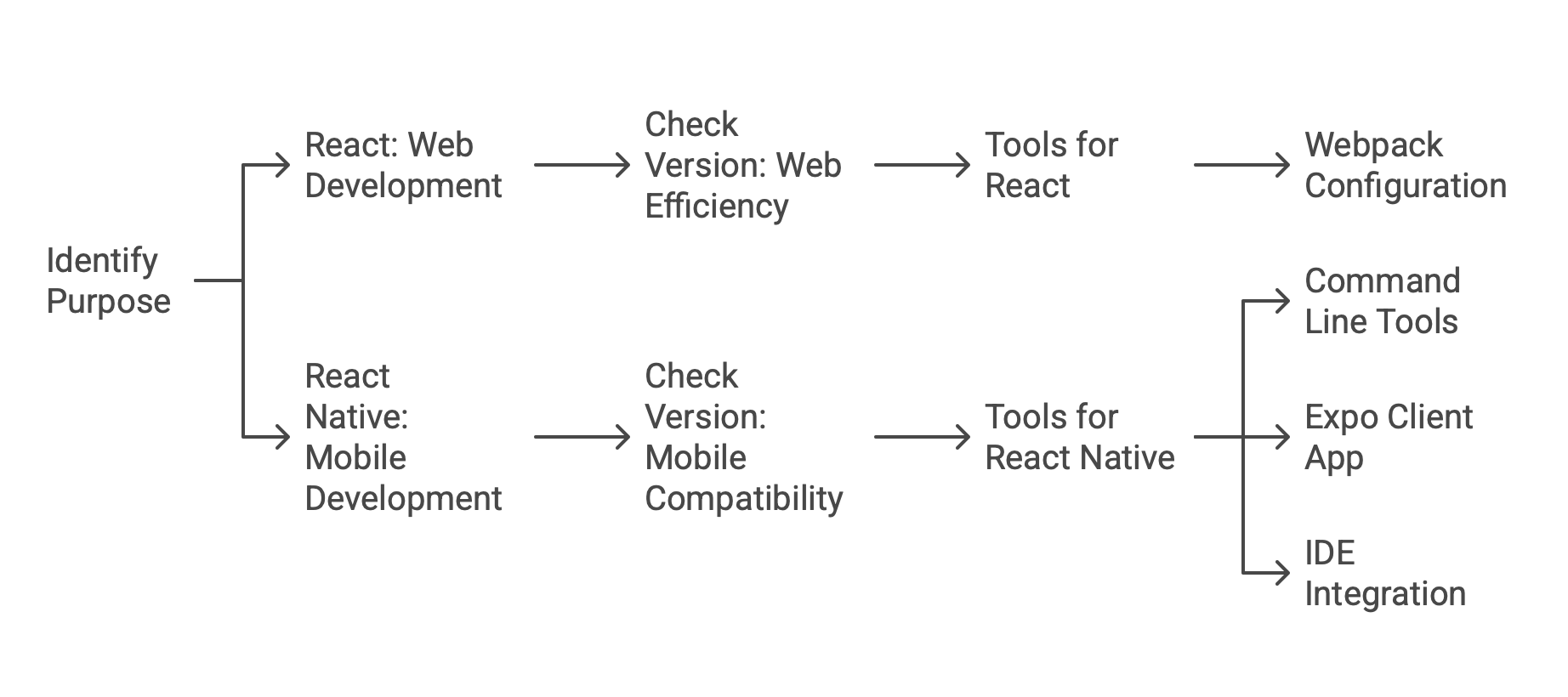
IDENTIFYING REACT NATIVE VERSION IN YOUR PROJECT
To find the RN version in your project, go to your project directory.
- Open the
package.json
file. This file is vital. It lists all dependencies and their versions. Look for "react-native" within the dependencies section. If you prefer command-line tools, run this command:npm list react-native
. - This will display the installed release directly in your console. For those using Yarn, a similar approach can be taken with
yarn list --pattern react-native
.
Another way to confirm your version is to check your JavaScript code. Import RN and log its version using console.log(ReactNative. Version);
. These methods make it easier to track changes in mobile app development. They also ensure compatibility.
TOOLS FOR CHECKING RN VERSION
To check the RN version, several tools can streamline the process, assuming React Native is already installed on your local development system. One of the most straightforward methods is using the command line.Simply navigate to your project directory and run react-native --version
. This immediately reveals your version. Another handy tool is npm or yarn. Run npm list react-native
or yarn list react-native
in your terminal. It will show the installed package version in your project's dependencies.
Tool/Method | Description |
---|---|
react-native --version | Run this command in the terminal to display the globally installed RN CLI version. |
npm list react-native | Use this command in the project directory to show the version of ReactNative installed in the project. |
package.json | Check the dependencies section of your project's package.json file for the RN version. |
Expo CLI | If using Expo, run expo diagnostics to display the RN version used in your project. |
DevTools/Console Log | Add console.log(require('react-native/package.json').version); to your code to log the version. |
The Expo client app shows version details on its dashboard. This makes it easy for users to check quickly. Integrating an IDE like Visual Studio Code can also help. It has plugins that show package versions in the editor. This allows for easy reference while coding.
WHY IS IT IMPORTANT TO KNOW YOUR REACT VERSION?
Knowing your React-version is essential for several reasons. First, it helps you know the available features and functions. Each release can introduce new hooks, optimizations, or changes that enhance development experience. Compatibility issues often arise when integrating third-party libraries or tools. Knowing the version lets you ensure all parts of your stack work well together. Performance improvements are another critical factor. Newer versions of React.js usually have improvements.
They make apps faster. Understanding your version provides insight into potential security vulnerabilities. Older releases may not receive updates or patches, leaving applications exposed to risks. The use of JSX, is a key feature of React, and its capabilities may change across versions. Tracking your React-version helps you plan upgrades and cuts disruptions to ongoing projects.COMPATIBILITY ISSUES WITH DIFFERENT REACTJS VERSIONS
Compatibility issues can arise when working with different versions of ReactJS. Each release may add features, optimizations, or breaking changes. They may affect your app's performance and stability. When upgrading to a newer version of React.js, check the release notes for conflicts. Certain functionalities might be deprecated or altered significantly. This can lead to unexpected bugs if your code relies on older APIs.
Third-party libraries often lag behind in compatibility updates. An outdated library with a new version of React.js may cause integration issues. They could derail your project timeline. Testing thoroughly after each upgrade is crucial. Automated tests catch bugs early. They ensure your app works as expected in different scenarios and environments.
PERFORMANCE IMPROVEMENTS IN NEWER REACT JS VERSIONS
Newer versions of React.js bring significant performance enhancements that developers eagerly adopt. A key improvement is concurrent rendering. It lets React work on multiple tasks at once. This means smoother user experiences and faster updates. React also optimizes how it processes changes with features like automatic batching. Instead of updating the DOM after every state change, React groups them. This makes re-rendering more efficient. This reduces unnecessary renders and boosts overall application speed.
Hooks have revolutionized component logic management. They enable fine-tuned control over rendering behavior while promoting clean code architecture. The performance benefits, revealed in benchmarks with each release, including improved load times and reduced bundle sizes, depend on the specific React-version installed on your system.Smaller bundles lead to quicker downloads for users. This is vital in mobile environments with limited bandwidth. These advancements keep React at the forefront of web development. They make apps run fast and efficiently, giving users a smooth experience.
HOW DO I UPDATE MY REACT VERSION?
Updating your React-version is easy, but it needs careful attention. If you're using create react-app, the process is seamless. To update, follow these steps:- Run the command
npm outdated
to see which packages need updates. This will give you an overview of outdated dependencies, including React itself. - To update React and its packages, run
npm install react@latest react-dom@latest
. This ensures you're getting the most recent stable versions. After updating, it’s wise to test your application thoroughly.
Remember that breaking changes can occur between major releases. Check the release notes for any changes that might affect your app before updating.
UPDATING REACT APPLICATION IN A CREATE-REACT-APP PROJECT
Updating a ReactJS application in a create react-app project is straightforward. Start by opening your terminal and navigating to the project directory.
- Start by running
npm install react@latest react-dom@latest
in your project directory. This command fetches the latest stable versions. - For projects not based on create react-app, you’ll need to manually adjust
package.json
. Also open the file and change the release number for both React and React DOM according to your needs. - After saving, run
npm install
oryarn install
. Before making these updates, consider dependency compatibility. Check if third-party libraries rely on specific React-versions. They might conflict later.
MANUALLY UPDATING REACT VERSION IN PACKAGE.JSON
To manually update the React-version in your project, you’ll need to edit the package.json
file directly.
- Start by opening this file in your preferred text editor.
- Locate the dependencies section and find entries for both "react" and "react-dom." You’ll see their current version numbers listed next to them.
- Simply replace these existing numbers with the new versions you wish to use. It’s essential to choose a compatible version based on your project's requirements.
- After saving the changes, use the following command:
npm install
oryarn install
, in your terminal. This command updates yournode_modules
folder with the specified React-versions.
WHAT SHOULD I CONSIDER BEFORE UPDATING CURRENT VERSION OF REACT APP?
Before updating your app, it's wise to assess dependency-compatibility. Ensure all libraries and plugins used in your project support the latest version of React. Outdated dependencies may cause conflicts or unexpected behavior. Next, examine breaking changes introduced in the latest React release notes. These changes can impact functionality and require code adjustments. Understanding these nuances will save you headaches during the update process. Consider testing thoroughly before going live with any updates. Utilize staging environments to catch issues early on without affecting end users. Watch for performance gains in newer versions. But, be cautious of their interaction with legacy code. A good plan will keep things stable. It will let you use upgrades effectively.
CHECKING DEPENDENCY COMPATIBILITY
When updating your React-version, checking dependency compatibility is crucial. Different libraries might rely on specific versions of React.js. If there’s a mismatch, it can lead to unexpected behavior in your application. Start by reviewing the documentation for each library you use.
Many maintainers will specify which versions of React.js are supported. This helps ensure that everything works smoothly after an update. Another approach is to use tools like npm-check-updates or yarn upgrade-interactive, referencing the version variable in the previous command of the process. These tools show what needs attention and can help upgrade while checking compatibility. Be mindful when updating. Sometimes, it's better to stick with older versions if they work well within your project. However, staying abreast of updates for the best React framework for building your application is also important. Always test thoroughly before deploying any changes. It's vital when many dependencies are linked to your main framework.BREAKING CHANGES BETWEEN REACT-VERSIONS
When updating React, it’s essential to be aware of breaking changes between versions. These can significantly affect your application's functionality. React releases often add new features. They may also remove old methods or change existing APIs.
- Backward Compatibility Issues: Code that functioned correctly in older versions might break after an upgrade.
- Example: React Hooks: React 16.8's introduction of Hooks significantly altered state management and lifecycle methods.
- Refactoring Required: Developers using class components in previous React-versions needed to refactor their code to accommodate Hooks.
- Importance of Release Notes: Carefully reviewing release notes for each update is crucial to understand potential breaking changes and necessary adjustments.
They provide insights into what has changed and suggest migration strategies if necessary. Always test your app after an upgrade. It may have issues from the breaking changes. Do this before deploying it live.
HOW CAN I CHECK REACT-RELATED PACKAGE VERSIONS?
To check React-related package versions, start by examining your package.json
file. This file lists all dependencies in your project. Look for entries like "react," 'react-dom," or any other relevant packages. Another straightforward method is using the command-line. You can run the below command npm list react
, which will display the installed version of React. If you're working with Yarn, simply use yarn list --pattern react
. For a broader view of all dependencies and their versions, execute npm outdated
. This command shows you what’s currently installed versus what's available.
VERIFYING REACT DOM VERSION
To verify the version of react_dom in your project, start by checking your package.json-file. This file contains all dependencies for your React application. Look under the "dependencies" section.
- You should see an entry for react_dom along with its version number listed next to it. If you prefer a command-line approach, simply run
npm list react-dom
oryarn list react-dom
. The output will display the installed version directly in your terminal. - Another method is to access it through JavaScript-code. Just import reactDOM and log its version using
console.log(ReactDOM.version)
.
However, this last method may not work. Not every library has a way to check its version. You should track your libraries. It will avoid issues and ensure compatibility.
CHECKING REACT-SCRIPTS VERSION IN CREATE-REACT-APP PROJECTS
If you work with a Create React App project, you must know both the React and react scripts versions. This package is vital. It provides your app's build tools and config.
You won't have to manually set up complex configs. To check the react scripts version, look in your project's package.json-file. Locate the "dependencies" section. There, you'll find an entry for react-scripts. It will look like this: json "react-scripts": "^X. X. X"
The number next to it is the installed version.
Alternatively, run this command in your terminal: bash npm list react scripts
. It will show the installed version and its dependencies.
Aligning the versions of React and react scripts can help. It can boost performance and cut compatibility issues. Regularly checking these versions helps maintain a healthy development environment. It ensures updates are seamless. This lets you use new features and improvements as they become available.